Idle Game Maker Developer Console Guide
The developer console can be a crucial tool to working with and debugging any game in IGM, however, to my knowledge there is no comprehensive guide of all of the special features IGM has to be used in the developer console. These features are probably unintended, as they are largely a consequence of how IGM's backend is structured. I intend to help with this guide into many of the features that you can use to help debug your game with IGM's often obtuse and unhelpful error messages, underscoring its overall lack of decent debugging features.
To open the console do ctrl+shift+i (Win) or cmd+opt+i (Mac) or right-click on the webpage and click Inspect. Once this is done, click over to the Console tab. Here's what it looks like in Chrome:
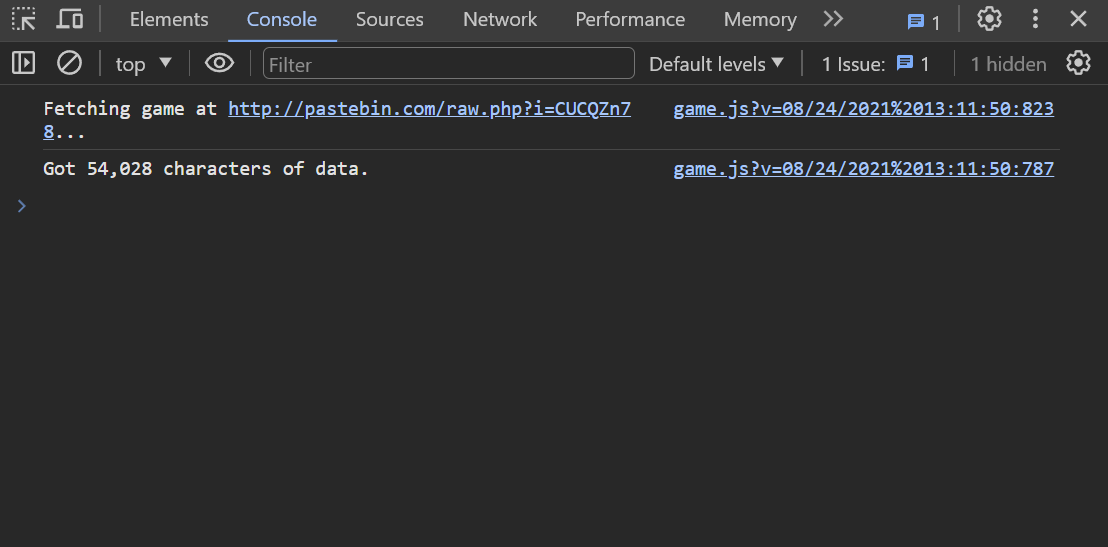
Before you can do much with the developer console, you need to make sure the console environment is 'player' instead of 'top'. The dropdown is shown as follows, although it may look a bit different to you due to extensions you have installed:
Entering script into the console is simple; you just type and hit enter. The console will remember whatever you typed in previously, for example:
G.things is a list of every thing in your game, along with all of their properties. You may also see specific types of things by using one of the following:
- G.res
- G.buttons
- G.buildings
- G.upgrades
- G.items
- G.achievs
- G.shinies
- G.boxes
These all work basically the same, they just have different types of things within them. When something works for G.things, it should also work for each of the categories. Your list of things will look something like this:
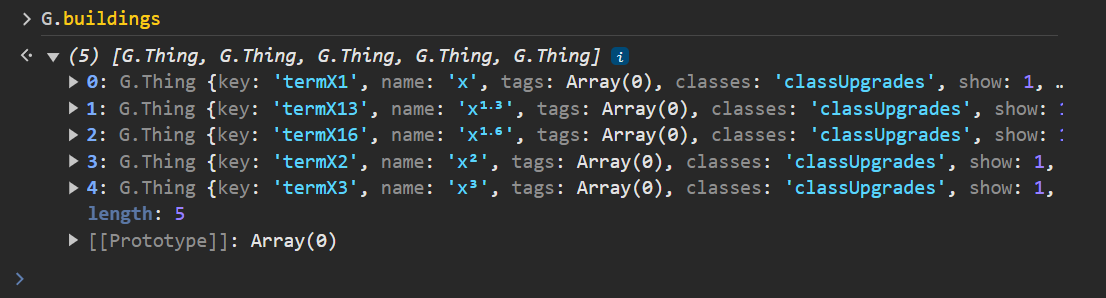
Since G.things is a list, we can access a thing at a certain index, for example:

G.Thing is the proper container of all data associated with a thing. Each type of thing has different properties associated with it. It will look something like this(in the case of a resource):
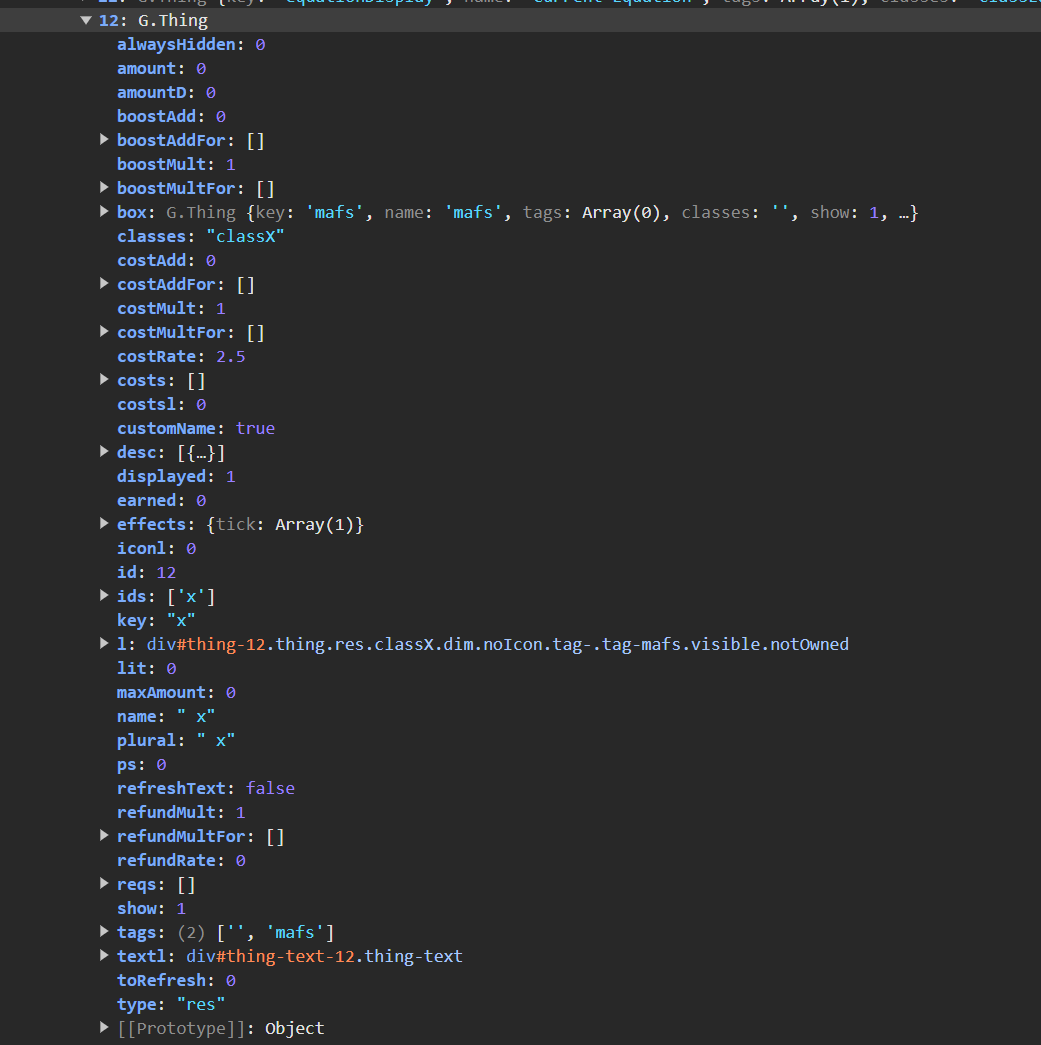
Looks intimidating! But don't worry, most of it is pretty self-explanatory. I'll go through each type of thing property-by-property and explain what the important ones are. Feel free to ctrl+f to find what you need.
To edit a property, double-click on the property value, change the value, and then press enter, like this:
Properties of all things:
- key
: The thing key as set by the thing declaration in your code
- type
: The type of your thing. Can be res, button, building, upgrade, itemType, achiev, or shiny. A thing can also be a box, although these are a special case that we will cover later.
- name
: The name of the thing that is displayed if the thing is shown
- id
: A numeric thing id that is the thing's position in G.things
- desc
: The thing's description as set in your code, formatted to HTML
- effects
: A list of effects the thing carries out
- classes
: A list of CSS classes the thing has
- tags
: A list of all of a thing's tags
- reqs
: A list of the requirements that must be met in order for the thing to show. If the list is empty, the thing has no requirements.
- show
: 1 if the thing is shown, 0 if the thing is hidden
- alwaysHidden
: 0 if the thing does not have the always hidden attribute, 1 otherwise
- box
: The container the thing is placed in the Layout portion of your code
Properties of resources:
- amount
: The current amount of the resource
- maxAmount
: The maximum amount of the resource the save file has
- earned
: The cumulative total amount of the resource you have earned across the save file
Properties of buttons:
- clicks
: The number of times the button has been clicked
- effects.click
: The effects that occur on click
- icon
: The button's icon
Properties of buildings:
- amount
: The number of that building currently owned
- costs
: A list of the building's costs
- costRate
: The cost increase percentage as set by your Settings section
- maxAmount
: The maximum amount of that building owned in the save file
- icon
: The building's icon
Properties of upgrades:
- owned
: 1 if the upgrade is owned, 0 otherwise
- costs
: A list of the upgrade's costs
- icon
: The upgrade's icon
Properties of items:
- base
: The itemType that the item is a member of(itemType does not have any unique properties outside of icon)
Properties of achievments:
- owned
: 1 if the achievment is owned, 0 otherwise
Properties of shinies:
- clicks
: The number of times the shiny has been clicked
- costs
: The cost of the shiny
- dur
: The amount of time the shiny will stay visible
- durMult
: A multiplier for the duration
- freq
: The base amount of time between when the shiny will spawn
- freqV
: The variation of the frequency
- icon
: The shiny's icon
- moves
: A list defining how the shiny should move
- timeLeft
: How much time is left until the shiny will spawn again
Properties of boxes:
Boxes are a special type of thing, and as such, don't share many of the otherwise universal properties within different things. A box is less of a thing itself and more of a container of things, defined in the Layout section of your code. Boxes aren't all that important to work with in the console in my experience, but I'll go ahead and list some of their properties anyway.
- children
: The things or type of thing the box should contain
- l
: The HTML div the box creates
- parent
: The box that the box is in
- showCosts
, showIcons
, showNames
: Properties to apply to the things the box contains
The G.tick method is the code the game calls in order to advance the game tick every second. You yourself can call G.tick() in the developer console in order to advance the game by a certain number of ticks. For example, for(let i=0; i<1000; i++) {G.tick();}
causes G.tick to be called 1000 times, effectively skipping 1000 ticks into the future.
You can also speed up time 2x by doing the following: setInterval(function() {G.tick()}, 1000);
. This works by calling G.tick every second(aka 1000 ms), and you can further speed up time by lowering the time between the ticks. For example, if you wanted to 3x speed up time, you would do this: setInterval(function() {G.tick()}, 500);
, or you could run setInterval(function() {G.tick()}, 1000);
twice.
G.clear()
: Wipes the current save file and saves, the same thing as clicking the Wipe button in the Settings panel
G.load()
: Instantly loads the last save
G.save()
: Instantly saves the current game
G.getSaveData()
: Returns the current save's data
In the console, you can define any amount of Javascript, including a set of custom functions. I have created a set of helper functions that I've found to be very useful for debugging in IGM. To use them, copy-paste the following into your terminal, same as you would G.things: